TimothE
TimothE is the engine we created for the Group Game Development Project and Work Base Simulation Module Assignment, we then additionally created a game called "Timothy Needs To Die" using this engine.
Learn MoreView On Github
Project Overview
TimothE is the engine we created for the Group Game Development Project and Work Base Simulation Module Assignment, we then additionally
created a game called "Timothy Needs To Die" using this engine.
My Contributions
- > Engine - UI Elements
- > Engine - Colliders
- > Game - Farming System
The team for this project consisted of more people and therefore forced more teamwork, the features inside of the engine which I worked on also had other members of the team working on them, for example, I alone did not make all the UI elements for the game. However, the farming system within the game was solely done by me.
Code Snippets / Screenshots
UI Elements
As we worked as a team a lot of my contributions were helping others with debugging and making sure all the UI elements could work with each other. The
main UI element I worked on solo were the buttons.
As our game mimicked the way in which Unity worked with adding components to game objects I made the button a component as it can be added to a UI GameObject.
Due to the nature of the module a lot of the work was with others and therefore I do not want to claim their code as my own within the portfolio.
class Button : public Component
{
public:
Button(GameObject* parent);
~Button();
COMPONENT_STATIC_TYPE(Button_Type);
// Component Methods
void OnStart() override;
void OnUpdate() override;
void OnEnd() override;
void DrawEditorUI() override;
// OnClick management
void AddClickEvent(void (*function)());
void RemoveClickEvent(void (*function)());
// Getter & Setter
bool IsHovering() { return _isHovering; }
bool IsClicked() { return _isClicked; }
private:
bool _isHovering;
bool _isClicked;
// OnClick Calls
vector _onClickCalls;
};
class CircleColliderComponent : public ColliderBase
{
public:
CircleColliderComponent(GameObject* owner);
void OnStart() override;
void OnUpdate() override;
void OnEnd() override;
float GetRadius() const { return _radius; }
glm::vec2 GetPosition() const { return _position; }
private:
float _radius;
glm::vec2 _position;
};
class BoxColliderComponent : public ColliderBase
{
public:
COMPONENT_STATIC_TYPE(Collider)
// Constructor & Deconstructor
BoxColliderComponent(GameObject* parent);
~BoxColliderComponent();
// Component Methods
void OnStart() override;
void OnUpdate() override;
void OnEnd() override;
virtual void DrawEditorUI() override;
// Getters & Setters
bool IsEnabled() { return _isEnabled; }
void SetEnabled(bool state) { _isEnabled = state; }
Rect* GetCollisionRect() { return _boxCollider; }
virtual bool SaveState(IStream& stream) const override {
Component::SaveState(stream);
WriteFloat(stream, _boxCollider->xPos);
WriteFloat(stream, _boxCollider->yPos);
WriteFloat(stream, _boxCollider->width);
WriteFloat(stream, _boxCollider->height);
WriteInt(stream, (int)_isEnabled);
return true;
}
virtual bool LoadState(IStream& stream) override {
Component::LoadState(stream);
_boxCollider = new Rect(
ReadFloat(stream),
ReadFloat(stream),
ReadFloat(stream),
ReadFloat(stream));
_isEnabled = (bool)ReadInt(stream);
return true;
}
private:
bool _isEnabled;
Rect* _boxCollider;
// Editor UI
bool* _editorIsEnabled;
};
Colliders
For the game we require colliders to detect collisions the code to the left shows the header files for the two different colliders I created for the engine.
These colliders are utilised in all of the collisions for the game and allows us to have functioning buttons and a functioning game. I utilised the colliders
within the button class and within the farming system within the game.
However as it was a group project we can see someone added a saving system to the engine which is not my code.
Farming System
We had development student plan what the game would be and as a programmer I worked on some of the elements they wanted within their game,
the main system I worked on was farming, the idea was that farming would be the main way the player would generate income to buy upgrades and better tools
The way I created a farming system was to have a class called "CropPlot" a class called "PlantedCrop" and finally a manager called "FarmlandManager",
The crop plot would be created when the user hoes the ground, the planted crop would be planted onto this crop plot, and the manager would manage all the crops.
This is the main controller to allow the player to plant crops, plant seeds, remove seeds, harvest grown crops, and to tell the crops when to grow to the next stage.
Finally to allow for easier editing of crops values we use CSV files so I had to create a method for loading in these CSVs so the crops know how long they should wait till being fully grown
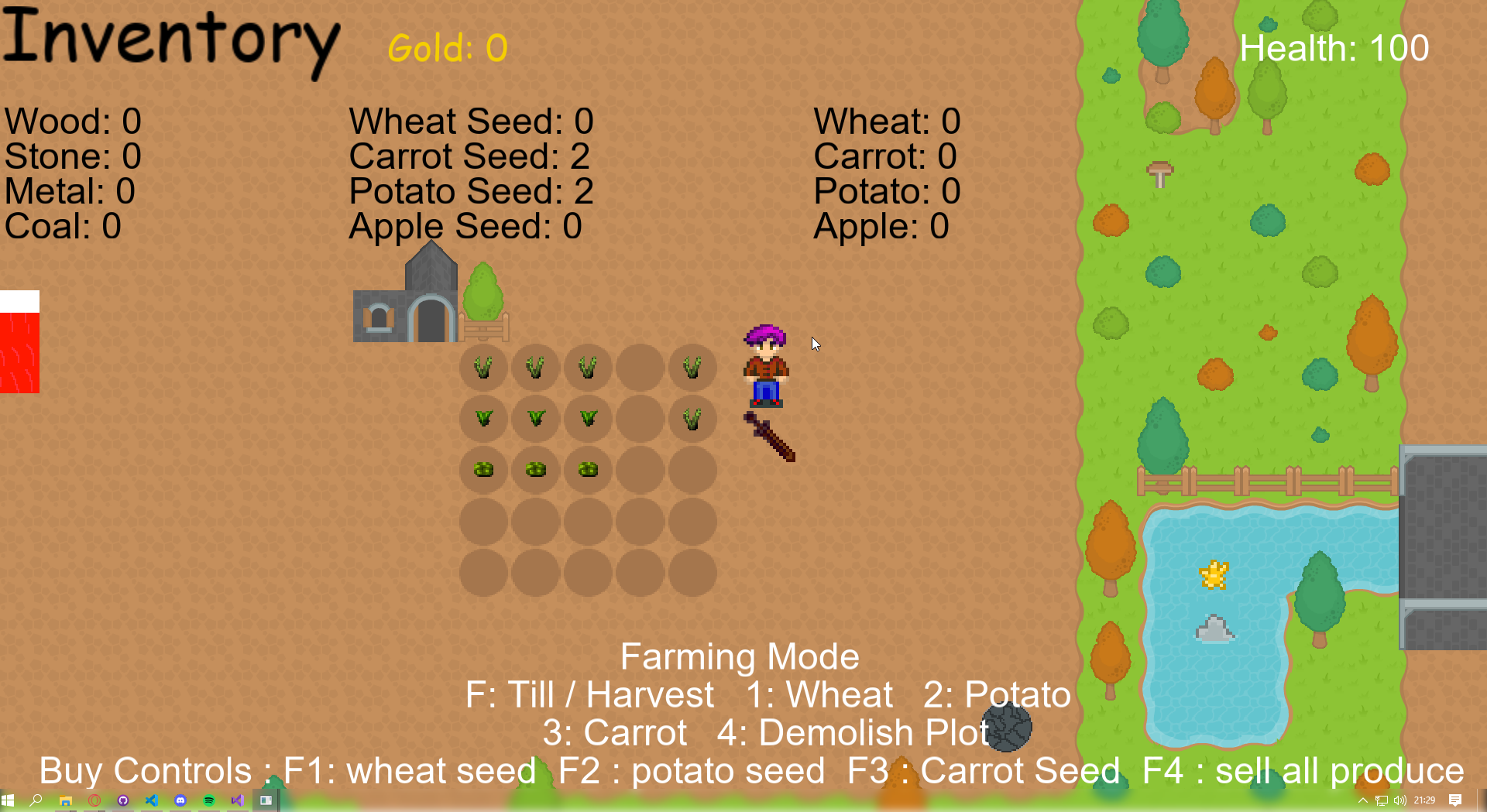
The way in which crops are brought was not the intention for the final product however due to time constraints, a team member was unable to add a shop system
therefore I had to implement a simple way to get a final product for submission.
I am happy to go into further detail for this or any projects on my portfolio if needs be.